Given the root of a binary tree, invert the tree, and return its root.
Example 1:
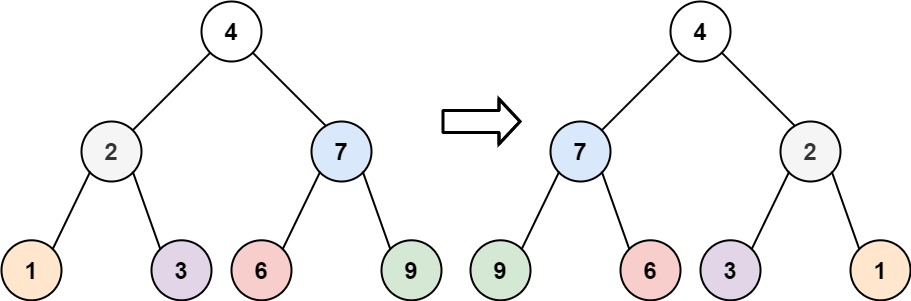
Input: root = [4,2,7,1,3,6,9]
Output: [4,7,2,9,6,3,1]
Example 2:
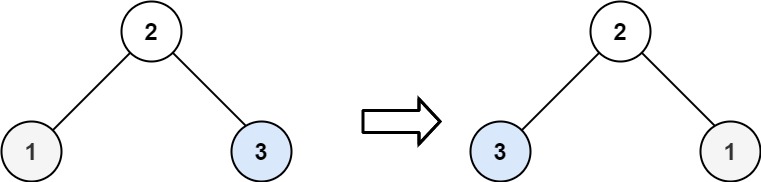
Input: root = [2,1,3]
Output: [2,3,1]
Example 3:
Input: root = []
Output: []
Constraints:
The number of nodes in the tree is in the range [0, 100].
-100 <= Node.val <= 100
翻转二叉树。
给你一棵二叉树的根节点 root ,翻转这棵二叉树,并返回其根节点。
思路
是一道不会做,会写 homebrew 也枉然的题。题干即是题意。例子如下,即层层遍历,把左右子树交换。
两种思路,分别是层序遍历(BFS)和深度遍历(DFS)。
复杂度
时间O(n)
空间O(n)
代码
BFS
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43
|
class Solution { public TreeNode invertTree(TreeNode root) { if (root == null) { return root; }
Queue<TreeNode> queue = new LinkedList<>(); queue.offer(root); while (!queue.isEmpty()) { int size = queue.size(); for (int i = 0; i < size; i++) { TreeNode cur = queue.poll(); TreeNode temp = cur.left; cur.left = cur.right; cur.right = temp; if (cur.left != null) { queue.offer(cur.left); } if (cur.right != null) { queue.offer(cur.right); } } } return root; } }
|
JavaScript实现
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23
|
var invertTree = function(root) { if (!root) return root; let queue = [root]; while (queue.length) { let current = queue.shift(); if (current === null) continue; swap(current); queue.push(current.left); queue.push(current.right); } return root; };
var swap = tree => { let temp = tree.left; tree.left = tree.right; tree.right = temp; return tree; };
|
DFS
Java实现
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36
|
class Solution { public TreeNode invertTree(TreeNode root) { if (root == null) { return root; } helper(root); return root; }
private void helper(TreeNode root) { if (root == null) { return; } TreeNode temp = root.left; root.left = root.right; root.right = temp; helper(root.left); helper(root.right); } }
|
JavaScript实现
1 2 3 4 5 6 7 8 9 10 11 12
|
var invertTree = function(root) { if (root === null) return root; let left = invertTree(root.left); let right = invertTree(root.right); root.left = right; root.right = left; return root; };
|